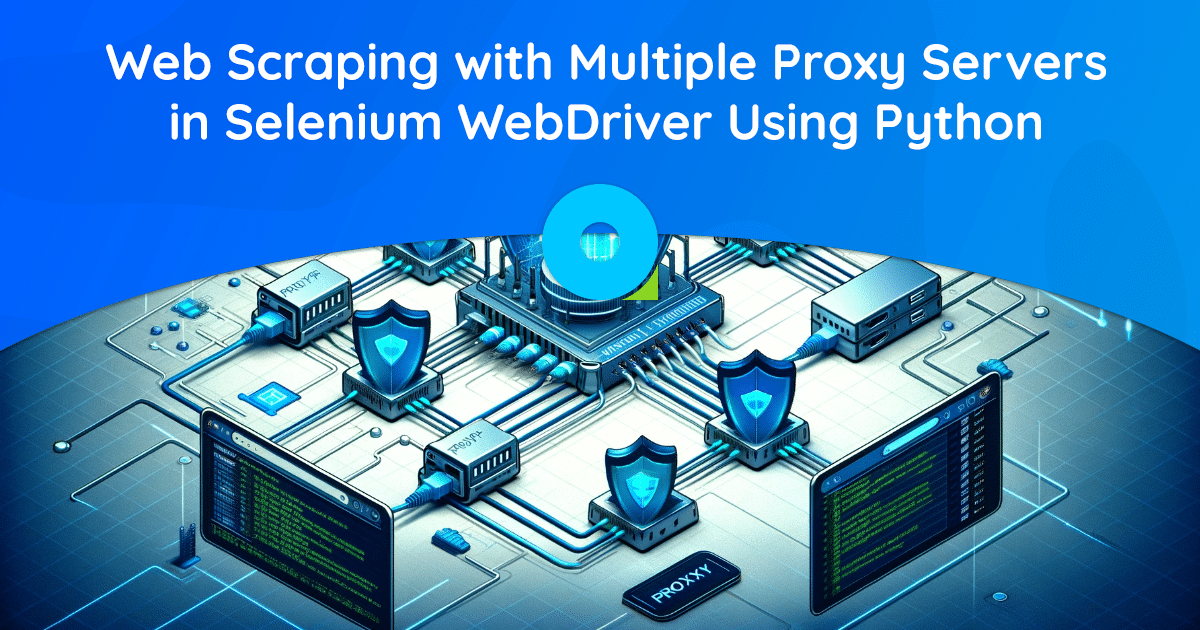
Web scraping is a technique used to extract large amounts of data from websites where the data is not readily available for download. This method is particularly useful in various scenarios, including market research, price comparison, real estate listing aggregation, weather data monitoring, social media analysis, and more. Here’s a more detailed look into its applications and importance:
- Market Research and Competitive Analysis: Businesses use web scraping to gather data from competitor websites, such as product pricing, descriptions, and customer reviews. This information is crucial for competitive analysis, pricing strategies, and understanding market trends.
- Price Comparison: Web scraping is widely used in the e-commerce industry for price comparison. By scraping data from various online retailers, companies can compare prices and offer competitive rates to their customers.
- Lead Generation: Sales and marketing teams scrape web data to gather contact information from business directories or social media platforms for lead generation purposes.
- SEO and Digital Marketing: Web scraping helps in SEO monitoring by extracting data on keyword rankings, backlinks, and content from competitors’ websites. This data is invaluable for optimizing SEO strategies.
- Real Estate and Property Listings: In the real estate sector, scraping is used to collect data from property listing sites, providing valuable information on market prices, property details, and historical trends.
- News Aggregation and Monitoring: Media and news agencies use web scraping to track online news stories and social media posts, helping them stay updated with the latest trends and events.
- Social Media Analysis: Analyzing social media data through web scraping helps in understanding public opinion, brand sentiment, and emerging trends.
- Financial Market Analysis: In finance, web scraping is used to gather data from financial portals for stock market analysis, monitoring exchange rates, and economic indicators.
- Academic Research: Researchers in various fields use web scraping to collect data sets from multiple sources for analysis, studies, and experiments.
- Product Development and Innovation: Companies scrape user reviews and feedback from various platforms to gain insights into customer preferences, helping in product development and innovation.
However, web scraping often leads to challenges such as IP address blocking or being served outdated data, mainly because websites want to control their data and prevent overloading of their servers. This is where proxies come into play. Proxies, by masking the user’s IP address and routing requests through different servers, help in avoiding bans and rate limits imposed by websites. They enable users to scrape data more efficiently and anonymously, ensuring uninterrupted access to the required data.
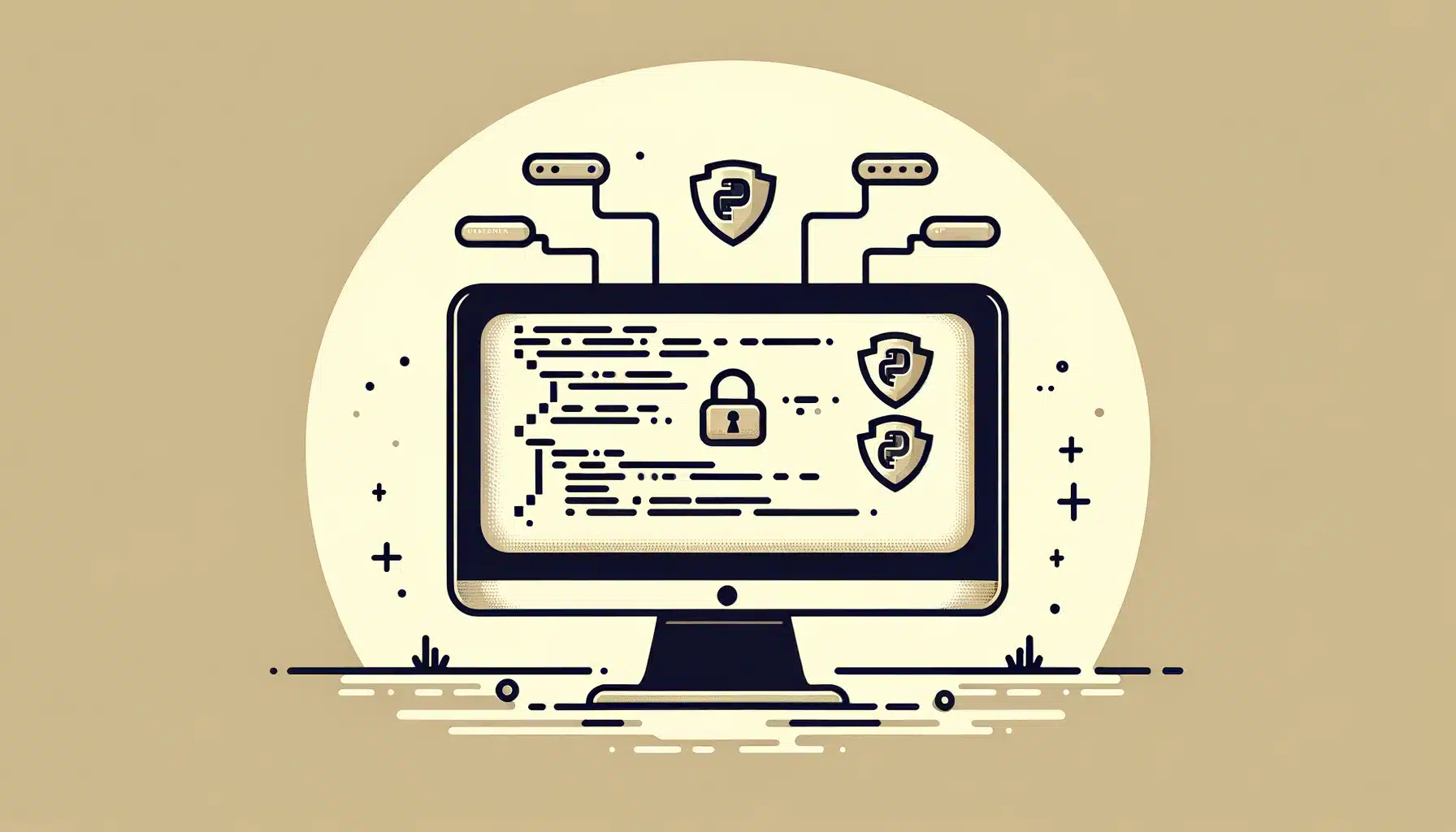
Proxies
Proxies serve as the middleman (server P) to contact a target server (server A), routing the response back to the user. They are especially useful in scenarios where users need to mask their identity or simulate multiple clients accessing a website, thereby circumventing IP-based restrictions imposed by web services.
Setting Up the Environment
Begin by installing the http-request-randomizer
package using Python’s package manager pip:
pip install http-request-randomizer
Gathering and Managing Proxies
With http-request-randomizer
, you can dynamically collect a list of proxies:
from http_request_randomizer.requests.proxy.requestProxy import RequestProxy
req_proxy = RequestProxy()
proxies = req_proxy.get_proxy_list()
Proxy Details
Examine the IP address and country of origin for each proxy in the list:
print(proxies[0].get_address()) # '179.127.241.199:53653'
print(proxies[0].country) # 'Brazil'
Integrating Proxies with Selenium WebDriver
Selection and Setup
Select a proxy from the list for use with Selenium WebDriver. For instance:
PROXY = proxies[0].get_address()
print(PROXY) # '179.127.241.199:53653'
Configuring Firefox
Configure the Firefox WebDriver to utilize the selected proxy:
from selenium import webdriver
webdriver.DesiredCapabilities.FIREFOX['proxy'] = {
"httpProxy": PROXY,
"ftpProxy": PROXY,
"sslProxy": PROXY,
"proxyType": "MANUAL"
}
driver = webdriver.Firefox(executable_path="path_to_geckodriver")
Configuring Chrome
Similarly, set up the Chrome WebDriver:
from selenium import webdriver
webdriver.DesiredCapabilities.CHROME['proxy'] = {
"httpProxy": PROXY,
"ftpProxy": PROXY,
"sslProxy": PROXY,
"proxyType": "MANUAL"
}
driver = webdriver.Chrome(executable_path="path_to_chromedriver")
Verifying IP Anonymity
Verify the proxy’s effectiveness by checking the IP address:
driver.get('https://oneproxy.pro/ip-address/')
Iterative Proxy Usage: Enhancing Web Scraping Efficiency
Iterative proxy usage is a crucial strategy in web scraping, particularly when dealing with websites that have stringent request limits or anti-scraping measures. Here’s a more detailed breakdown of this process:
- Rotating Proxies: Use a rotation system for proxies to distribute requests across multiple IP addresses. This practice reduces the likelihood of any single proxy being banned due to excessive requests. By rotating proxies, you mimic the behavior of multiple users accessing the website from different locations, which appears more natural to the target server.
Here’s an example of Python code to rotate proxies using thehttp-request-randomizer
library, ensuring requests are distributed across multiple IP addresses:
from http_request_randomizer.requests.proxy.requestProxy import RequestProxy
from selenium import webdriver
import time
# Initialize proxy manager
req_proxy = RequestProxy()
proxies = req_proxy.get_proxy_list()
def get_driver_with_proxy(proxy_address):
options = webdriver.ChromeOptions()
options.add_argument(f'--proxy-server=http://{proxy_address}')
driver = webdriver.Chrome(chrome_options=options, executable_path="path_to_chromedriver")
return driver
# Function to rotate proxies
def rotate_proxies(proxies, url, num_requests=10):
for i in range(num_requests):
proxy = proxies[i % len(proxies)].get_address()
driver = get_driver_with_proxy(proxy)
driver.get(url)
print(f"Using proxy: {proxy}")
time.sleep(2) # Adjust sleep time as needed
driver.quit()
# URL to scrape
target_url = "https://example.com"
rotate_proxies(proxies, target_url, num_requests=50)
This script sets up a proxy rotation system for web scraping using Selenium and http-request-randomizer
. It distributes requests across multiple IP addresses, mimicking natural user behavior and reducing the risk of bans. Adjust the num_requests
and time.sleep
values as needed for your specific use case.
- Request Management: Determine the request limit of each website you scrape. Websites often have a threshold for how many requests an IP can make in a given period before being blocked. Use each proxy for a number of requests that’s safely below this limit.
- Session Management: After using a proxy for its allocated number of requests, close the Selenium WebDriver session. This step is essential to clear cookies and session data, further reducing the risk of detection.
- Efficient Switching: Develop a system to switch proxies smoothly without significant downtime. This can involve pre-loading proxies or using a proxy pool where a new proxy is immediately available once the current one has reached its limit.
- Error Handling: Implement robust error handling to detect when a proxy is blocked or fails. The system should automatically switch to the next proxy without manual intervention to maintain the scraping process’s continuity.
Optimizing for Speed with Local Proxies
Using local proxies, or proxies from the same country as the target website, can significantly enhance the speed of web scraping. Here’s an extended look at this approach:
- Latency Reduction: Local proxies usually offer lower latency compared to international ones, as the data doesn’t have to travel as far. This results in faster load times and more efficient scraping.
- Relevance of Data: For certain types of scraping, like gathering local news or market prices, local proxies might provide more relevant data, as some websites serve different content based on the user’s location.
- Balance Between Speed and Diversity: While local proxies can be faster, they limit the diversity of your proxy pool. A smaller pool increases the risk of exhausting available proxies, especially if the target site has strict rate limiting or ban policies.
- Considerations for Local Proxy Selection: When selecting local proxies, it’s essential to assess their quality, speed, and reliability. The ideal scenario would involve a substantial pool of local proxies to ensure both speed and a lower risk of bans.
- Fallback Strategies: In cases where local proxies are limited, have a fallback strategy involving proxies from neighboring countries or regions with similar network performance. This ensures that the scraping process continues smoothly even if local proxies are exhausted or temporarily unavailable.
A well-planned proxy strategy, combining both iterative usage and the optimization of local proxies, can significantly enhance the efficiency and speed of your web scraping endeavors while minimizing the risk of detection and IP bans.
Conclusion
Employing multiple proxies in Selenium WebDriver with Python presents a sophisticated solution for effective and anonymous web scraping. This approach not only helps in circumventing IP bans but also maintains a seamless data extraction process. However, users should be aware of the potential variability in proxy reliability and speed.
For those seeking a more robust and reliable solution, considering a premium proxy provider like OneProxy is advisable. OneProxy offers a vast range of high-quality proxies that are known for their speed, stability, and security. Utilizing such a premium service ensures consistent performance, minimizes the risk of being blocked, and offers a wider selection of geolocations for your scraping needs. Although it comes with a cost, the investment in OneProxy can significantly enhance web scraping efforts, particularly for professionals and organizations requiring high-volume and efficient data extraction.
Incorporating OneProxy into your web scraping strategy with Selenium WebDriver elevates the overall efficiency and effectiveness, providing a seamless experience even in the most demanding data extraction tasks.