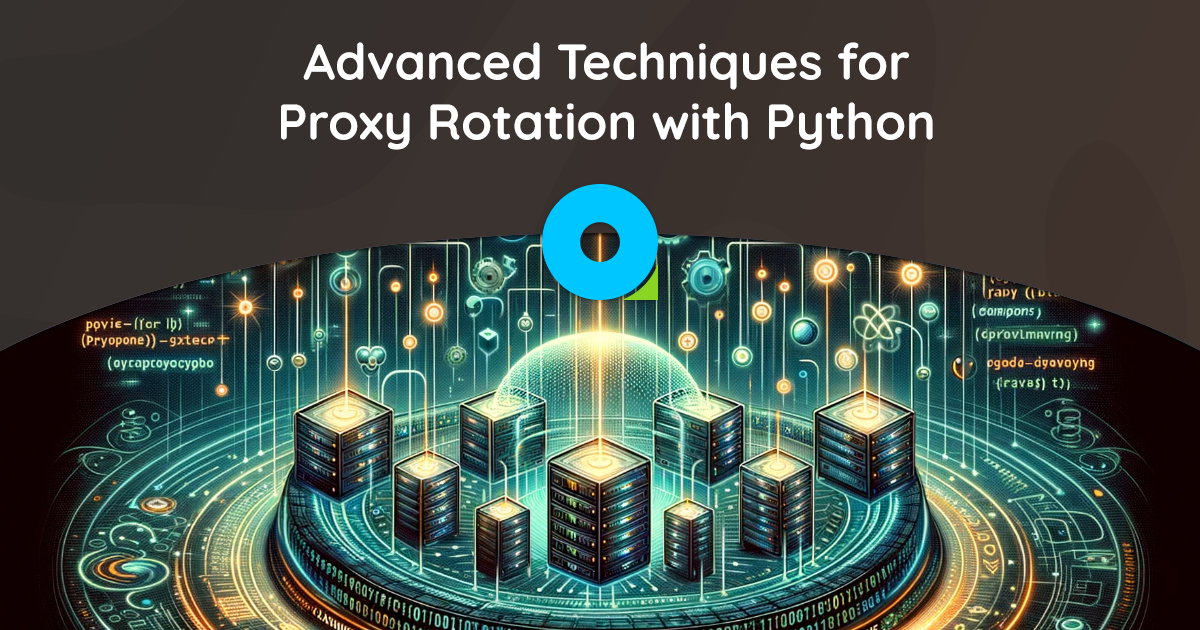
Creating an efficient proxy rotation mechanism is essential when dealing with large-scale web scraping or data mining tasks. While the early stages of web scraping projects or minimal-scale crawls might suffice with a basic setup, the real challenge arises when scaling up. To mitigate risks such as IP blocking and to ensure the robustness of your scraping infrastructure, utilizing a sophisticated proxy rotation system becomes imperative.
For such purposes, the use of a professional proxy service provider like OneProxy becomes invaluable. With a diverse pool of data center proxy servers, such services can vastly enhance the reliability and efficiency of your scraping tasks.
Below, we delve into the development of a more advanced proxy rotator using Python and Beautiful Soup, leveraging the services from OneProxy for optimal results.
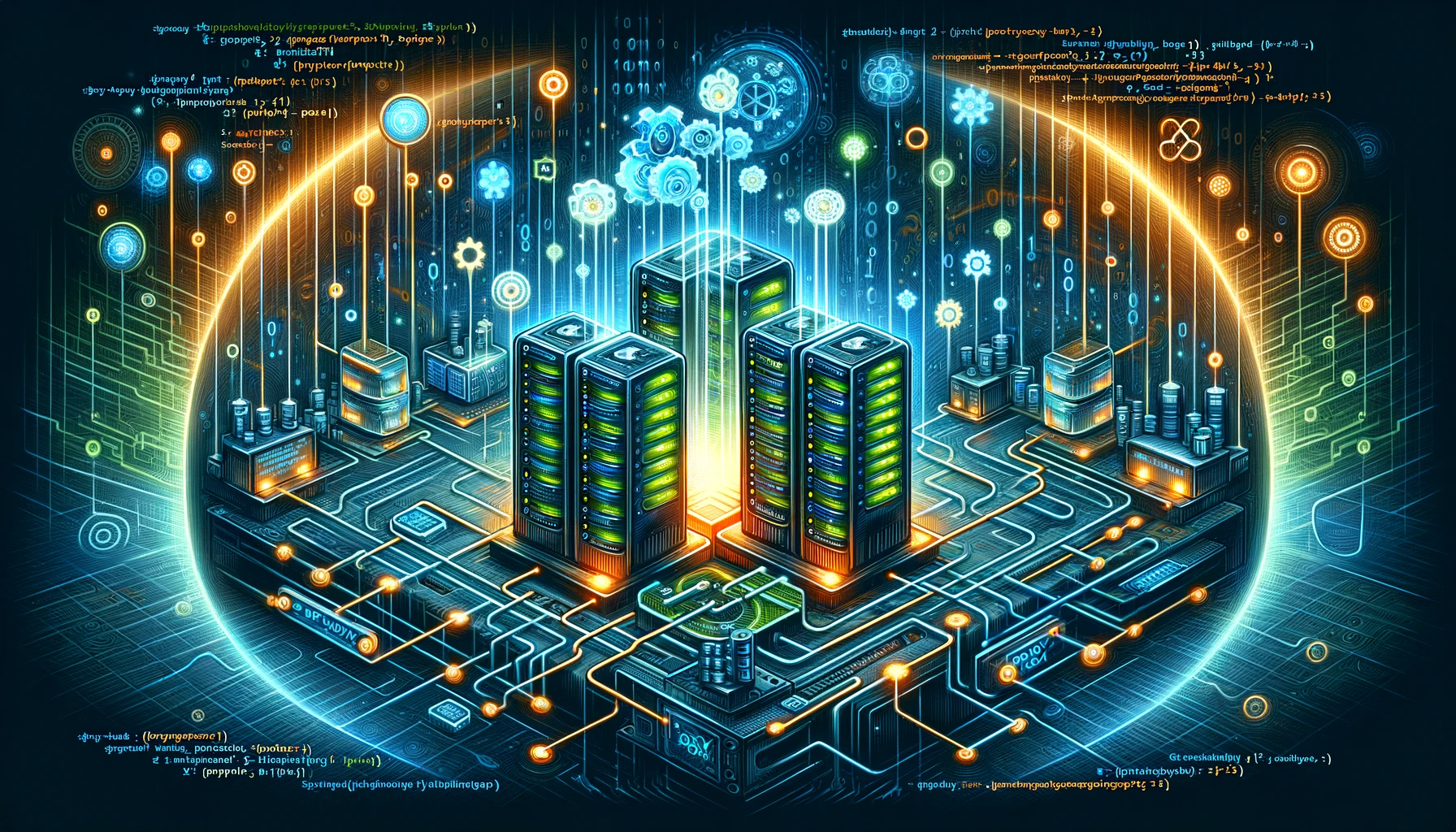
Preliminary Setup
Before you begin, ensure that you have Beautiful Soup and the requests
library installed in your Python environment. These tools will enable you to parse HTML content and manage HTTP requests easily.
Our proxy rotation script will fetch public proxies from OneProxy’s free proxy pool, which can be accessed at OneProxy Free Proxy List. This list is updated regularly, offering a fresh set of proxies for various needs.
Basic Fetch Code
First, we need to establish the basic code to fetch the HTML content from the OneProxy’s free proxy list. We use a user-agent string to emulate a web browser, which helps in bypassing basic user-agent based bot detections.
# -*- coding: utf-8 -*-
from bs4 import BeautifulSoup
import requests
url = https://oneproxy.pro/free-proxy/
def fetch_proxies(url):
header = {
'User-Agent': 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_11_2) ' +
'AppleWebKit/601.3.9 (KHTML, like Gecko) Version/9.0.2 Safari/601.3.9'
}
response = requests.get(url, headers=header)
return response.content
This function simply retrieves the HTML content from the provided URL.
Parsing the Proxy List
The BeautifulSoup
library will parse the HTML content to extract the proxies. The proxies are typically listed within a table structure on the web page, identified by specific HTML tags and attributes.
def parse_proxies(html_content):
soup = BeautifulSoup(html_content, 'lxml')
proxy_table = soup.select_one('#proxy-list-table') # Replace with the correct ID
proxies = []
for row in proxy_table.select('tr'):
columns = row.select('td')
if columns:
ip, port = columns[0].get_text(), columns[1].get_text()
proxies.append({'ip': ip, 'port': port})
return proxies
Rotating Proxies
The following function orchestrates the proxy rotation by randomly selecting an available proxy from the fetched list:
from random import choice
def rotate_proxies(proxies):
if proxies:
return choice(proxies)
else:
return None
Putting It All Together
Combining all the functions, the final script integrates proxy fetching, parsing, and rotation, providing a seamless proxy rotation system.
# -*- coding: utf-8 -*-
import requests
from bs4 import BeautifulSoup
from random import choice
# Functions previously defined: fetch_proxies, parse_proxies, rotate_proxies
proxies = [] # This will hold our list of proxies
def refresh_proxies():
global proxies
proxies = parse_proxies(fetch_proxies('https://oneproxy.pro/free-proxy/'))
def get_random_proxy():
if not proxies:
refresh_proxies()
return rotate_proxies(proxies)
# Main execution
refresh_proxies()
proxy = get_random_proxy()
print(proxy['ip'], proxy['port'])
Professional Scaling with OneProxy
For production environments where the scale extends to thousands of requests, free proxy pools may not suffice due to reliability and speed considerations. At this juncture, a rotating proxy service becomes essential.
OneProxy offers a robust solution with features such as:
- Global High-Speed Proxies: Millions of data center proxies worldwide ensure uninterrupted and rapid connections.
- Automatic IP Rotation: IP addresses are rotated seamlessly to prevent detection and bans.
- User-Agent String Rotation: Mimics requests from various web browsers and versions, enhancing the non-detectability of bots.
- CAPTCHA Solving: Integrates technology to solve CAPTCHAs automatically, thereby streamlining the scraping process.
With OneProxy, customers have triumphantly navigated the challenges of IP blocking, thereby streamlining their web data extraction processes.
OneProxy’s services are versatile and can be implemented in any programming language, catering to a wide array of projects and requirements.
Special Offer: Experience the power of professional proxy rotation with OneProxy. Get started with 50,000 requests at no cost